Software Defect Predictor
Using machine learning to predict possible defects in software patches.
For my SE465 - Software Testing, Quality Assurance, and Maintenance project, I used the WEKA machine learning library in Java to train a model that can predict the likelihood of a defect for a patch given the code change and metadata. Code changes are parsed into a bag-of-words model and used to train the model along with other metadata such as the number of files changed, and the time of submission. To achieve the best results, various models, such as Naive Bayes, Random Forest, and Alternating Decision Tree, were experimented with as well.
A Slack Bot written in Python and Flask.
This Slack Bot provides smarter custom response features than the default one on Slack. It is able to analyze past chat history and activate on a sequence of messages. It can also send multi-line messages and images. Webhooks are set up using the Flask server to listen to chat events. When certain conditions are fulfilled, the server will send a custom message using the Slack API.
A 2D turn-based strategy game developed using Java and Lightweight Java Game Library.
This game was made as the final project for my high school computer science course. I implemented the game logic, including unit interactions, action tracking, item system, and win/lose condition checking. In addition, I was also reponsible for the AI, which employed some concepts from game theories, such as Minimax.
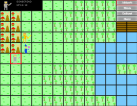
Customizable tiles, sprites, and maps with a turn-based chess-like battle system
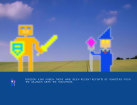
Animated cutscenes with a rich story
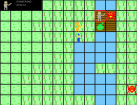
Smart AI that makes every battle a fun and exciting challenge
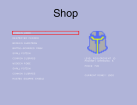
Shop and item system that introduces character customization and different ways to play
Scala Compiler
A compiler written in Scala, which compiled a subset of Scala into MIPS.
For my CS241e - Enriched Foundations of Sequential Programs course, I built a compiler that parsed a subset of Scala all the way down to MIPS assembly instructions. The compiler consisted of multiple parts, including:
- A simple semispace garbage collector which implemented Cheney's algorithm.
- A typer which performed type inference and validation.
- A parser using Cocke-Younger-Kasami algorithm that created a parse tree given the input tokens and the grammar.
- A maximal munch scanner which parsed input into tokens given a deterministic finite automaton.
- An assembler which transformed abstractions such as closures, control structures, variables, and expressions into machine code.
A Tetris game developed using Processing, a variation of Java, controlled using the TI Launchpad microcontroller.
This project was created as the final project for my SE101, Introduction to Methods of Software Engineering, course. The game logic is written in Java and played on a computer, while the TI Launchpad microcontroller is responsible for reading user inputs as well as showing the next block on its LCD display. I wrote most of the game logic as well as the serial communication protocol between the computer and the microcontroller.
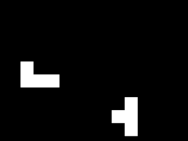
A screenshot of the Tetris game
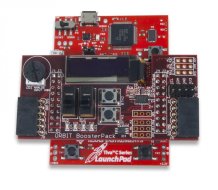
The TI Launchpad microcontroller with the orbit booster pack with a LCD screen and several buttons.
A gesture-controlled painting software developed in Java using the Myo armband.
This project was developed for the Deloitte Tech Exchange competition and Enghack. It uses Myo's Java library as well as the AWT library to map different hand gestures to different functions such as drawing, erasing, and adjusting color and thickness.
A Web Application built with Node.js, and Bootstrap, which uses the Watson news and language analytics API to calculate popularity and reception of products.
This project utilizes the News API of IBM Watson to search for articles about the product, and analyze the article using the Sentiment API. A rating is then assigned to the product by an algorithm within the program.
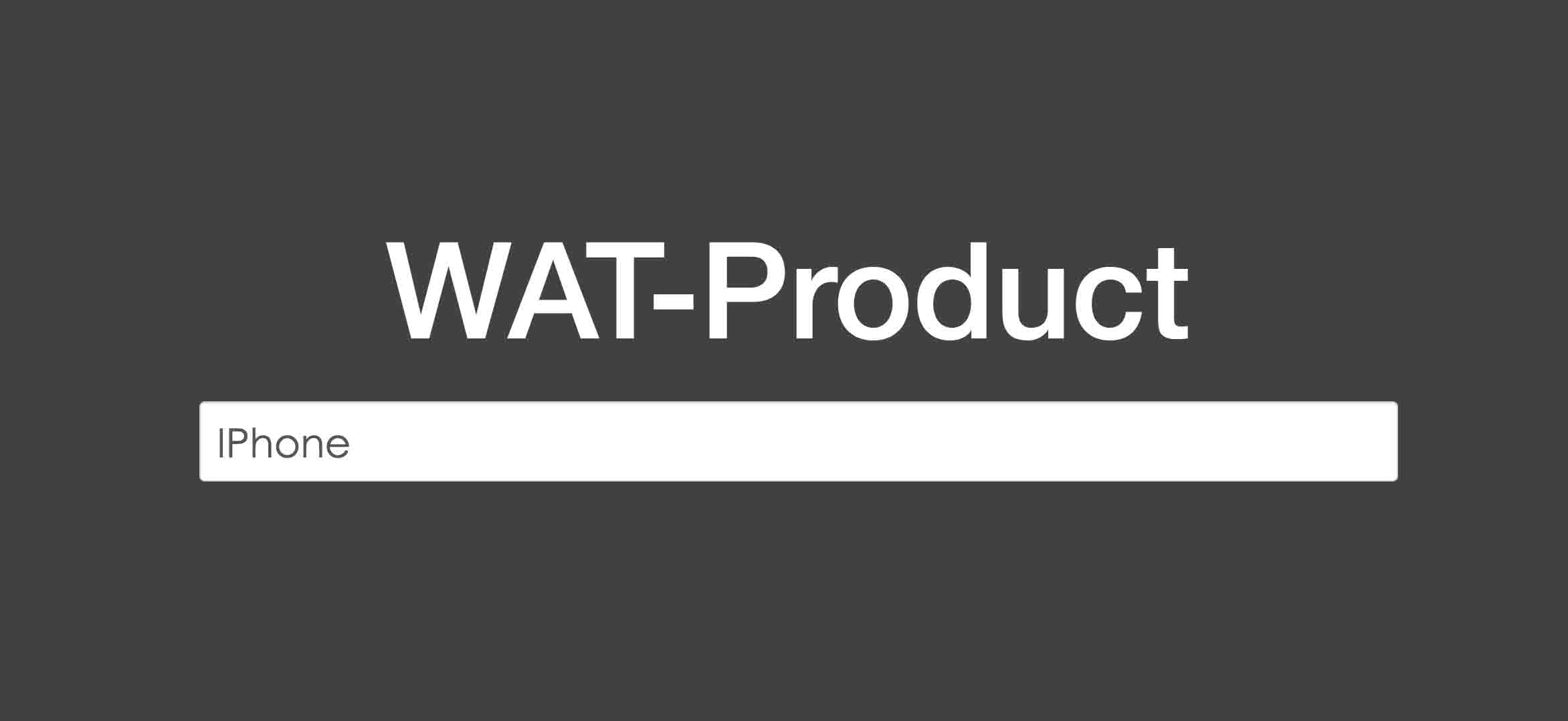
User enters a product
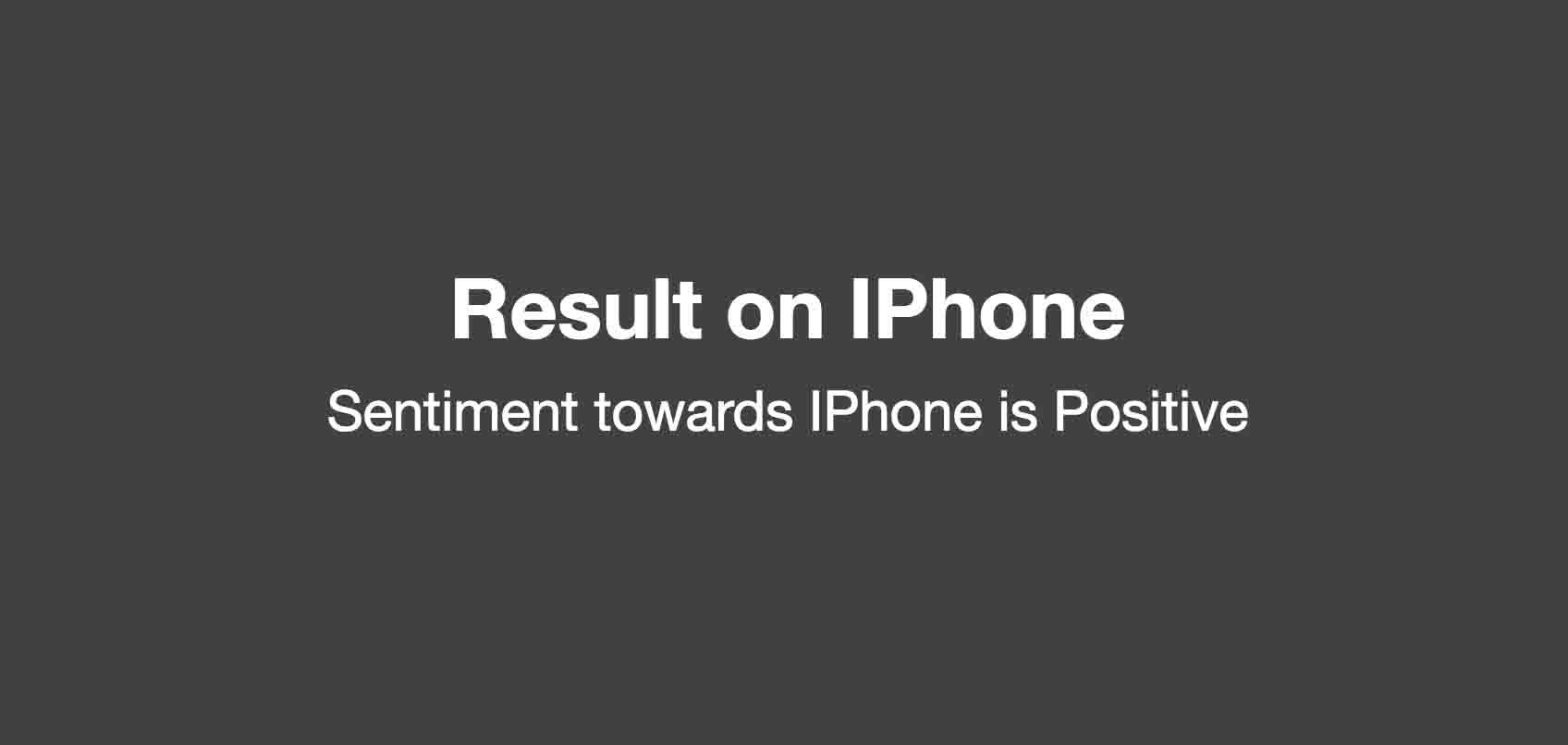
The server accesses the Watson API and determines whether the perception of the product is negative or positive